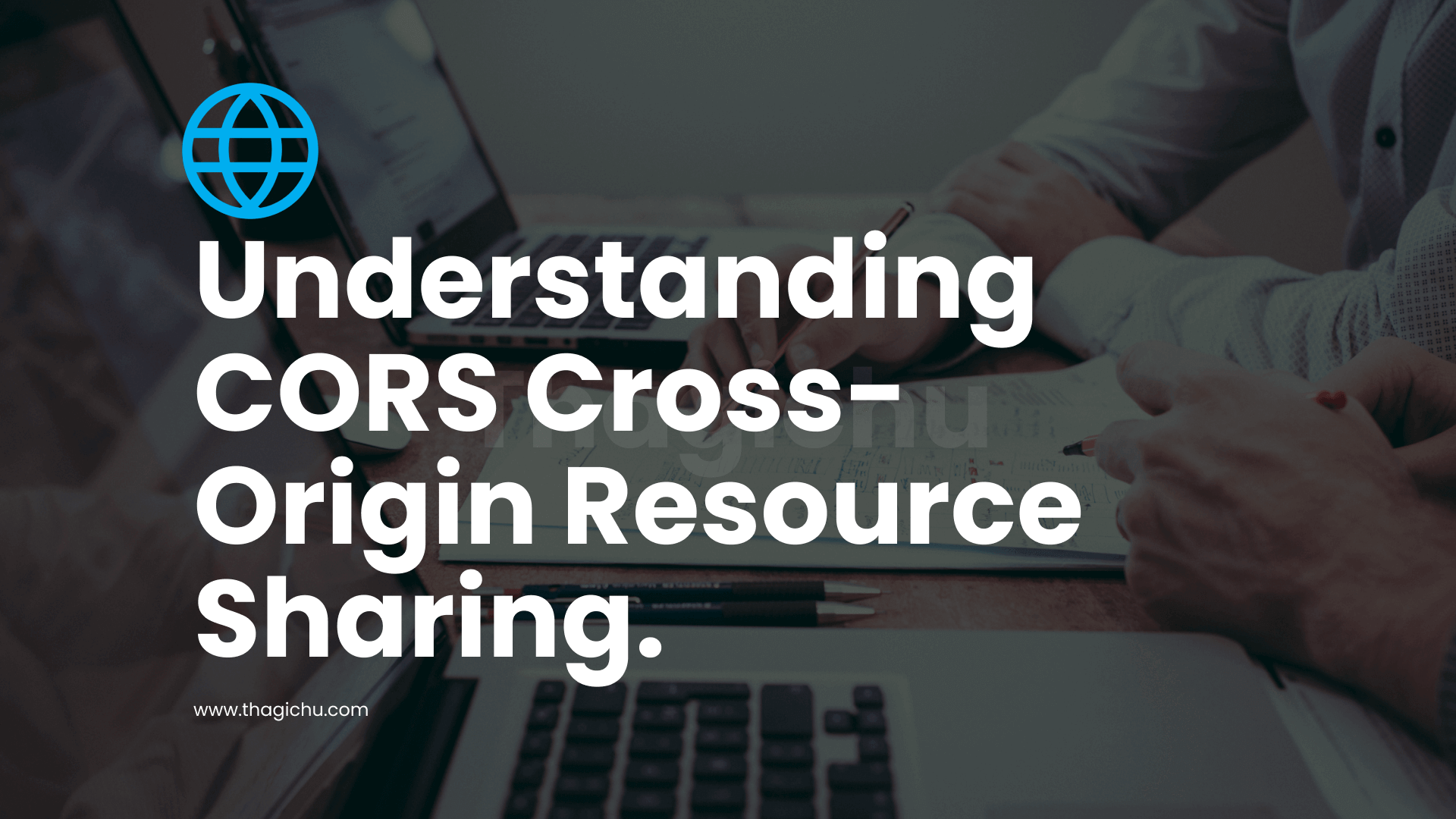
Understanding CORS (Cross-Origin Resource Sharing).
Published at Jun 6, 2022
Writen by Thagichu Anthony
Understanding CORS Cross-Origin Resource Sharing.
CORS stands for Cross-Origin Resource Sharing. It is a security feature implemented by web browsers to control web page access to resources hosted on other domains (i.e., origins). CORS is a crucial part of web security that helps prevent malicious websites from making unauthorized requests to a different domain on behalf of a user.
Here are the key aspects of CORS:
1.Same-Origin Policy (SOP):
By default, web browsers enforce the same-origin policy, which means that web pages can only make requests to resources on the same domain (same origin) from which the web page was served. This restriction is in place to prevent potential security vulnerabilities.
2.Cross-Origin Requests:
A cross-origin request occurs when a web page hosted on one domain makes a request for resources (e.g., data, images, scripts) hosted on a different domain.
3.CORS Headers:
To allow cross-origin requests in a controlled and secure manner, the server hosting the requested resource can include special HTTP response headers, known as CORS headers, in its responses. The most common CORS headers are:
- Access-Control-Allow-Origin:
Specifies which domains are allowed to access the resource. It can be set to a specific origin or to ”*” to allow any origin (though this should be used cautiously). i.e. server is running on http://localhost:8080 and the client is running at http://localhost:3000 from the server you can set “Access-Control-Allow-Origin”:“http://localhost:3000”
Express App
const express = require("express");
const app = express();
app.use((req, res, next) => {
res.setHeader("Access-Control-Allow-Origin", "http://localhost:3000");
// Other CORS headers like 'Access-Control-Allow-Methods', 'Access-Control-Allow-Headers', etc. can be added as needed.
next();
});
// Your routes and other middleware here
app.listen(8080, () => {
console.log("Server is running on port 8080");
});
Rust with Axum and Tower
use tower_http::cors::CorsLayer;
fn build_app() -> BoxRoute {
// rest of your app
use axum::http::method;
use tower_http::cors::{ CorsLayer};
let cors = CorsLayer::new()
.allow_origin("http://localhost:3000".parse::<HeaderValue>().unwrap())
.allow_methods([Method::GET, Method::POST, Method::PATCH, Method::DELETE])
.allow_credentials(true);
// rest of your app
// pass it on to your router
.layer(cors);
}
In each of these examples, we set the Access-Control-Allow-Origin header to “http://localhost:3000” to allow cross-origin requests from the client running at that origin. Make sure to configure any additional CORS headers or options that your application requires.
- Access-Control-Allow-Methods:
Specifies which HTTP methods (e.g., GET, POST, PUT, DELETE) are allowed when making cross-origin requests.
check example above
- Access-Control-Allow-Headers:
Lists the HTTP headers that are allowed in the request. e.i. you can choose to only accept certain headers or not
- Access-Control-Allow-Credentials:
Indicates whether the browser should include credentials (e.g., cookies, HTTP authentication) in the cross-origin request. The Access-Control-Allow-Credentials header is an HTTP response header used in Cross-Origin Resource Sharing (CORS) to indicate whether the browser should include credentials (e.g., cookies, HTTP authentication) in cross-origin requests. It is a security feature designed to control the sharing of sensitive user data between different origins.
Here are the key aspects of Access-Control-Allow-Credentials:
Values:
true:
If the server includes Access-Control-Allow-Credentials: true in its response, it signals to the browser that the cross-origin request can include credentials. This allows the browser to include cookies and HTTP authentication information in the request.
false:
If the server includes Access-Control-Allow-Credentials: false (or omits the header entirely), the browser will not include credentials in the cross-origin request.
Usage:
When you set Access-Control-Allow-Credentials: true, it indicates that the server is willing to accept credentials and authenticate cross-origin requests. This is particularly important when you have authentication mechanisms in place, and you want to make authenticated requests to a different domain. For example, if you have a web application that uses cookies for user authentication and you want to make AJAX requests to an API on a different domain, you would need to set Access-Control-Allow-Credentials: true on the API server.
Security Implications:
Enabling Access-Control-Allow-Credentials should be done with caution, especially if the credentials include sensitive information. It allows any website that can make a cross-origin request to access the user’s credentials for the target domain. It’s crucial to ensure that the target server is configured securely to handle cross-origin requests with credentials. Proper authentication and authorization checks should be in place to protect sensitive data.
Preflight Requests:
When Access-Control-Allow-Credentials is set to true, preflight requests (HTTP OPTIONS requests) sent by the browser during a cross-origin request will also include the Access-Control-Allow-Credentials header in their response to confirm that credentials are allowed.
Example of setting Access-Control-Allow-Credentials in a server’s response header:
HTTP/1.1 200 OK
Access-Control-Allow-Origin: http://localhost:3000
Access-Control-Allow-Credentials: true
In this example, the server is configured to allow cross-origin requests from http://localhost:3000 and to include credentials in those requests.
Keep in mind that the combination of Access-Control-Allow-Origin and Access-Control-Allow-Credentials headers must be set correctly to ensure that the browser behaves as expected.
Access-Control-Allow-Origin Header:
This header specifies which origins (domains) are allowed to make cross-origin requests to your server. It can be set to a specific origin (e.g., ”http://example.com”) or ”*” to allow any origin. The value of Access-Control-Allow-Origin must match the origin of the client making the request. If they don’t match, the browser will block the request due to the same-origin policy. It’s essential to set this header correctly to control which domains are allowed to access your resources. Access-Control-Allow-Credentials Header:
This header indicates whether the browser should include credentials (such as cookies and HTTP authentication) in cross-origin requests. If Access-Control-Allow-Credentials is set to true, the browser will include credentials if the following conditions are met: The client (origin) is allowed by Access-Control-Allow-Origin. The request is of a type that can include credentials (e.g., not a simple GET request). If Access-Control-Allow-Credentials is set to false or omitted, the browser will not include credentials, regardless of the Access-Control-Allow-Origin setting.
Note
If you want to allow cross-origin requests with credentials (e.g., cookies for authentication), you should set both Access-Control-Allow-Origin to the specific origin of the client and Access-Control-Allow-Credentials to true. If you don’t need credentials in cross-origin requests or you want to allow any origin to access your resources without credentials, you can set Access-Control-Allow-Origin to ”*” (wildcard) and omit or set Access-Control-Allow-Credentials to false.
- Access-Control-Max-Age:
Specifies how long the results of a preflight request (an initial request sent to check permissions) can be cached.
3.Preflight Request:
For certain types of requests (e.g., those with custom headers or non-standard HTTP methods), browsers send a preflight request (an HTTP OPTIONS request) to the server to check if the actual request is permitted. The server should respond to the preflight request with appropriate CORS headers.
CORS is essential for enabling cross-origin requests in web applications while maintaining security. It allows legitimate use cases like fetching data from third-party APIs or integrating different web services, but it requires careful configuration on the server side to prevent unauthorized access.
Developers need to configure their web servers to include the necessary CORS headers to enable or restrict cross-origin access as needed for their web applications.