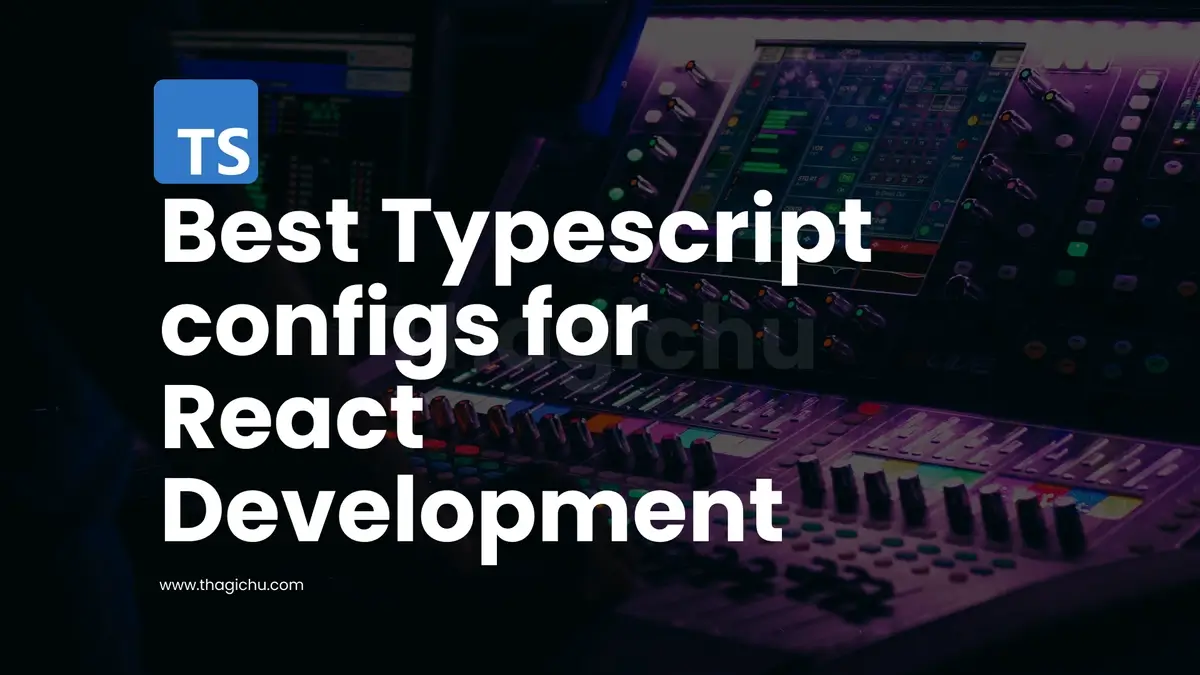
Typescript Setup for React Development
Published at Jun 6, 2022
Writen by Thagichu Anthony
Setting Up TypeScript for React Development
TypeScript is a statically typed superset of JavaScript that brings additional safety and tooling to your React development workflow. In this tutorial, we’ll walk you through the process of setting up TypeScript for React development step by step.
Prerequisites
Before getting started, make sure you have the following prerequisites installed on your machine:
Node.js and npm (Node Package Manager): You can download and install them from nodejs.org.
Step 1: Create a React App
We’ll start by creating a new React application using the create-react-app command-line tool. Open your terminal and run the following command:
npx create-react-app my-ts-react-app
This command creates a new React app named my-ts-react-app.
Step 2: Install TypeScript
Once your React app is created, navigate to its directory:
cd my-ts-react-app
To add TypeScript to your project, use the following command:
npm install --save typescript @types/node @types/react @types/react-dom @types/jest
This command installs TypeScript and type definitions for Node.js, React, ReactDOM, and Jest.
Step 3: Configure TypeScript
Create a tsconfig.json file in the root directory of your project. You can do this manually or by running:
npx tsc --init
Edit the tsconfig.json file to include the following options:
{
"compilerOptions": {
"target": "es5",
"lib": ["dom", "dom.iterable", "esnext"],
"allowJs": true,
"skipLibCheck": true,
"esModuleInterop": true,
"allowSyntheticDefaultImports": true,
"strict": true,
"forceConsistentCasingInFileNames": true,
"module": "esnext",
"moduleResolution": "node",
"resolveJsonModule": true,
"isolatedModules": true,
"jsx": "react-jsx",
"noEmit": true,
"incremental": true
},
"include": ["src/**/*.ts", "src/**/*.d.ts", "src/**/*.tsx", "src/**/*.js", "src/**/*.jsx"],
"exclude": ["node_modules"]
}
This tsconfig.json configuration is suitable for most React projects. It enables strict TypeScript checking, JSX support, and more.
Step 4: Rename Files to .tsx
Rename your React components from .js or .jsx to .tsx to indicate that they contain TypeScript code. For example, if you have a component named App.js, rename it to App.tsx.
Step 5: Update index.tsx
Open the src/index.tsx file and ensure it imports the React and ReactDOM libraries correctly:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App'; // Update this import if needed
import * as serviceWorker from './serviceWorker';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
Step 6: Start Your Development Server
Now that your project is set up with TypeScript, start your development server:
npm start
This command will start your React development server, and you can access your app at http://localhost:3000.
Step 7: Writing TypeScript in React
You can now start writing React components using TypeScript. TypeScript will provide type checking and autocomplete suggestions, making your development process smoother and less error-prone.
Here’s an example of a simple TypeScript-based React component:
import React from 'react';
interface Props {
name: string;
}
const Greeting: React.FC<Props> = ({ name }) => {
return <div>Hello, {name}!</div>;
};
export default Greeting;
With TypeScript, you can define prop types using interfaces or types to ensure the correct data types are used in your components.
Conclusion
Congratulations! You’ve successfully set up TypeScript for React development. TypeScript adds type safety to your React projects, making them more robust and maintainable. You can now enjoy the benefits of static typing while building your React applications.