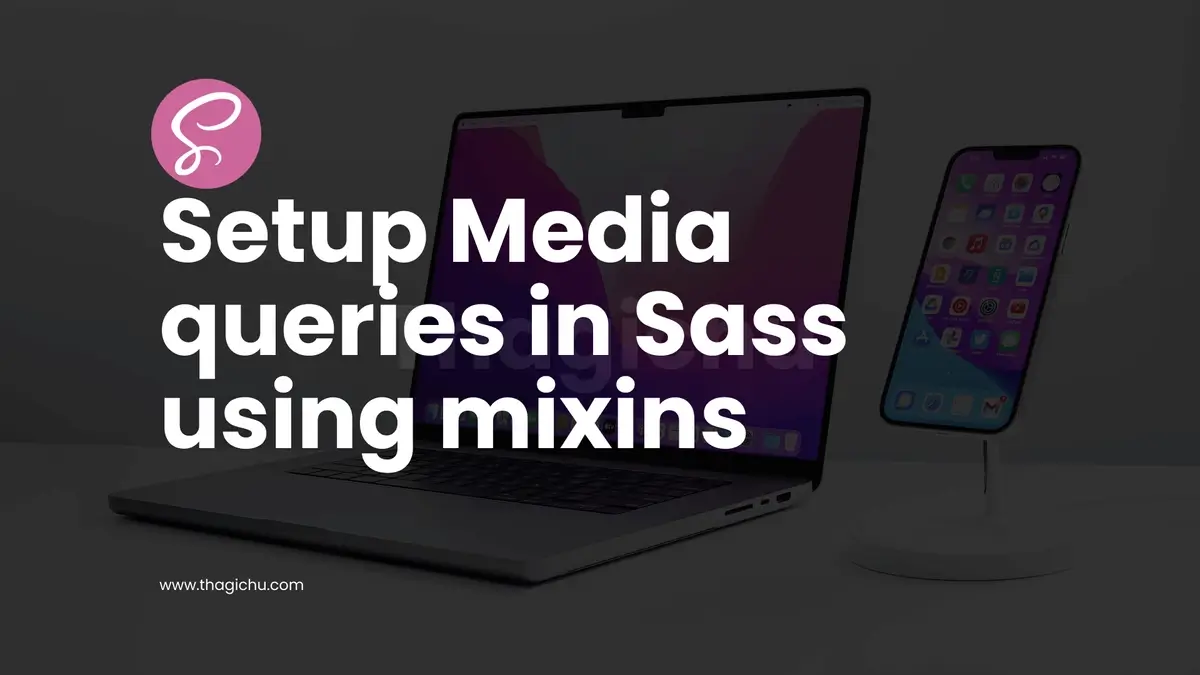
Media queries in SCSS
Published at Jun 6, 2022
Writen by Thagichu Anthony
Setting Up SCSS Mixins for Web Development
In the world of web development, writing clean and maintainable CSS code is crucial to building responsive and visually appealing websites. One powerful tool that can help you achieve this goal is SCSS (Sass). SCSS provides a way to write more organized and modular CSS through features like variables, nesting, and mixins. In this article, we’ll focus on SCSS mixins and how to set them up for your web development projects.
What Are SCSS Mixins?
Mixins in SCSS are reusable blocks of CSS code that can be included (or “mixed in”) within other SCSS rulesets. They allow you to define a set of CSS properties and then reuse them throughout your stylesheet, reducing redundancy and making your code easier to maintain.
Here’s a basic example of a SCSS mixin:
@mixin button-style {
border: 1px solid #3498db;
background-color: #3498db;
color: #ffffff;
padding: 10px 20px;
border-radius: 4px;
text-align: center;
text-decoration: none;
display: inline-block;
cursor: pointer;
}
In this example, button-style is a mixin that defines a basic button style. You can then include this mixin within your CSS rulesets to apply the same styling to multiple buttons.
Setting Up SCSS Mixins
Setting up SCSS mixins in your web development project is a straightforward process. Here are the steps to get started:
- Install SCSS If you haven’t already, you’ll need to install SCSS on your development machine. You can do this globally using npm (Node Package Manager) by running the following command in your terminal:
npm install -g sass
Create an SCSS File Create a new .scss file in your project directory or choose an existing one where you want to define your mixins. For example, you can create a file named mixins.scss.
Define Your Mixins Inside your .scss file, define your SCSS mixins. You can follow the example from earlier, where we created a button-style mixin. Feel free to create mixins for various styles and components in your project.
Import Mixins In your main SCSS file, where you compile all your styles, import the file containing your mixins using the @import directive:
@import 'mixins';
- Use Mixins Now that you’ve imported your mixins, you can use them within your CSS rulesets. To include a mixin, use the @include directive followed by the mixin’s name and any necessary parameters:
.button {
@include button-style;
In this example, the button-style mixin is applied to elements with the class .button.
- Compile SCSS to CSS To see your SCSS code in action, you need to compile it to standard CSS. You can do this using the sass command in your terminal. Navigate to your project’s root directory and run:
sass --watch your-main-stylesheet.scss:output.css
Replace your-main-stylesheet.scss with the name of your main SCSS file and output.css with the desired name for your compiled CSS file. The —watch flag will automatically recompile your SCSS to CSS whenever you make changes.
Benefits of Using SCSS Mixins
Using SCSS mixins in your web development projects offers several benefits:
Code Reusability: Mixins allow you to write code once and reuse it throughout your project, reducing duplication and making your codebase more maintainable.
Modularity: Mixins promote a modular approach to styling. You can create mixins for specific components or design patterns, making it easier to manage your styles.
Consistency: Mixins ensure consistent styling across your website, as the same styles can be applied to multiple elements without manual duplication.
Easy Maintenance: When you need to update a style, you can do so in one place (the mixin) rather than searching through your entire stylesheet for each instance.
In conclusion, SCSS mixins are a powerful tool for enhancing your web development workflow. By following the steps outlined in this article, you can set up and use SCSS mixins to streamline your CSS development process and create cleaner, more maintainable stylesheets for your web projects.