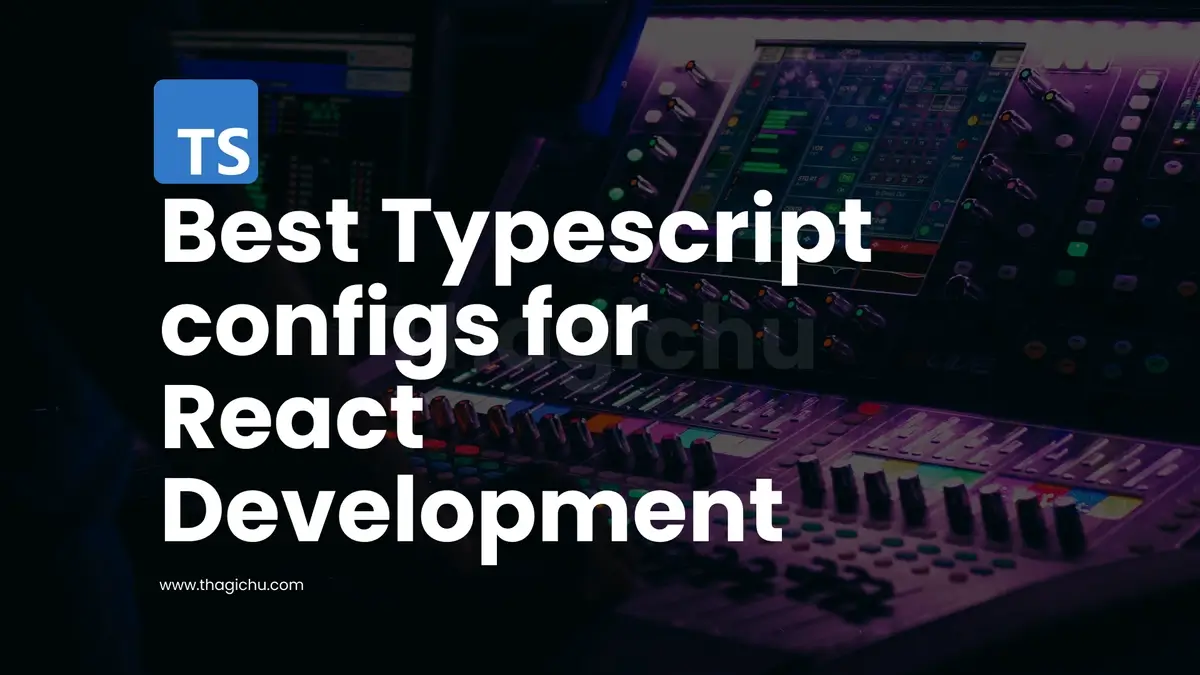
How to configure and setup sign up with Google using Google Provider
Published at Jun 6, 2022
Writen by Thagichu Anthony
Intro
Authentication is and has always been a difficult subject and one of the most commonly asked questions amoungest new and some senior developers especially when developing webapps. Next Auth offer an easier way to implement user Authentication for Next JS. In this article i will guide you on how to add Next Auth to an arleady existing Next JS
1. Install
npm install next-auth
yarn add next-auth
pnpm add next-auth
2. Added a Next JS Endpoint to handle Auth
create an endpoint under the api directory i.e. pages/api/auth/[…nextauth].js
// file: pages/api/auth/[...nextauth].js
import NextAuth from "next-auth"
export default NextAuth({
providers: [
],
})
3. Import Google Auth Provider
Add Google Auth Provider from providers/google
// file: pages/api/auth/[...nextauth].js
import GoogleProvider from "next-auth/providers/google";
4. Add Google Auth configuration to the list of providers
Add the imported GoogleProvider to the list of providers
// file: pages/api/auth/[...nextauth].js
...
providers: [
GoogleProvider({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET
})
]
...
5. Get Google API keys for your app
To get Google API Credentials: Google Developer Console
select Create Credentials
select OAuth Client id
if: To create an OAuth client ID, you must first configure your consent screen
click configure consent screen
select external
Fill out your App information
save and continue
go back to Create OAuth client ID
set Application Type as Web application
Authorized redirect URIs: http://localhost/api/auth/callback/google
copy your Client ID and Client Secret
6. Add the required env variables required for the Google Auth.
// file: .env
GOOGLE_CLIENT_ID= <Client ID>
GOOGLE_CLIENT_SECRET=<Client Secret>
7. Add Next Auth Provider to your Next JS App
Import the session provider from next auth and wrap the main Component .
// file: _app.js
...
import { SessionProvider } from "next-auth/react";
function MyApp({ Component, pageProps: { session, ...pageProps } }) {
return (
<SessionProvider session={session}>
<Component {...pageProps} />
</SessionProvider>
);
}
export default MyApp;
8. Using the Google Provider Example
// file: index.jsx
import { useSession, signIn, signOut } from "next-auth/react"
export default function Home() {
const { data: session } = useSession();
if (session) {
return (
<div >
Welcome user<br />
<button onClick={() => signOut()}>Sign out</button>
</div>
);
}
return (
<div>
Sign In with Google <br />
<button onClick={() => signIn()}>Sign in</button>
</div>
);
}
9. Creating an SSR protected page
// file: protectedpage.jsx
...
import { getSession } from "next-auth/react";
function protectedpage() {
return (
<div>
<h1>Protected Page</h1>
</div>
);
}
export async function getServerSideProps(context) {
const session = await getSession(context);
if (!session) {
context.res.writeHead(302, { Location: "/" });
context.res.end();
return {};
}
return {
props: {
user: session.user,
},
};
}
export default protectedpage;